Analog output instrument
The AnalogOut instrument provides multiple channels of analog output on devices that support it, such as the Analog Discovery and the Analog Discovery 2. It provides the functionality normally associated with a stand-alone arbitrary waveform generator.
Todo
This section is currently incomplete.
It lacks a detailed discussion of how all the settings work.
Important
The AnalogOut channels are designed to be capable of operating independently.
To that end, each AnalogOut channel has its own settings and state, and its behavior is fully independent from the behavior of the other analog output channels, unless explicitly commanded using the AnalogOut.masterSet()
method.
Because of this, each AnalogOut channel can be considered a fully independent instrument.
Using the analog output instrument
To use the AnalogOut instrument you first need to initialize a DwfLibrary instance. Next, you open a specific device. The device’s AnalogOut instrument can now be accessed via its analogOut
attribute, which is an instance of the AnalogOut
class.
For example:
from pydwf import DwfLibrary
from pydwf.utilities import openDwfDevice
dwf = DwfLibrary()
with openDwfDevice(dwf) as device:
# Get a reference to the device's AnalogOut instrument.
analogOut = device.analogOut
# Use the AnalogOut instrument: reset all output channels.
analogOut.reset(-1)
The AnalogOut channel state machine
Each AnalogOut channel is controlled by a state machine. As an output sequence is prepared and executed, the channel goes through its various states.
The current state of the channel is returned by the analogOut.status()
method, and is of type DwfState
.
The figure below shows the states used by the AnalogOut instrument and the transitions between them:
States of the AnalogOut instrument
The AnalogOut states are used as follows:
-
In this preparatory state, channel settings can be changed that specify the behavior of the channel in the coming output sequence. If the auto-configure setting of the device is enabled (the default), setting changes will automatically be transferred to the device. If not, an explicit call to the
analogOut.configure()
method is needed to transfer updated settings to the device.Once the channel is properly configured, an output sequence can be started by calling the
analogOut.configure()
with the start parameter set to True. This will start the first stage of the output sequence by entering theArmed
state. -
In this state the channel continuously monitors the configured trigger input. As soon as a trigger event is detected, the instrument proceeds to the
Wait
state. -
In this state, the analog output is driven according to the channel’s Idle setting. The duration of the wait state is configurable. Once this duration has passed, the channel proceeds to the
Running
state. -
In this state the channel drives its output according to its node settings. This continues until the run duration has been reached. The channel then proceeds to the Repeat state.
Repeat
Note
This is not a true state, in that there is no
DwfState
value that represents it. It is included here to explain the control flow of the AnalogOut channel state machine.When an output run is finished, the repeat count is decremented.
If, after decrementing, the repeat count is unequal to zero, more output must be produced. If the repeat trigger setting is True, the channel proceeds to the
Armed
state; in that case, a trigger is needed to start each of the output runs. If the repeat trigger setting is False, the channel proceeds immediately to theWait
state to start another output sequence; a trigger is only required before the very first output run.If, after decrementing, the repeat count did reach zero, the channel becomes idle and proceeds to the
Done
state.-
This state indicates that an output sequence has finished. In this state, the analog output is driven according to the channel’s Idle setting.
From this state, it is possible to go back to the
Ready
state by performing any kind of configuration, or to start a new output sequence.
AnalogOut channel nodes
AnalogOut channels are organized in nodes, which can be independently configured. A node represent either the primary non-modulated signal (the Carrier), or some form of modulation, like Amplitude Modulation (AM) or Frequency Modulation (FM). The output of each node varies over time according to its settings. The node outputs are combined to synthesize the signal that is driven onto the analog output channel via a DAC.
Note
Early versions of the library only implemented the Carrier signal and lacked modulation support. With the introduction of AM and FM modulation, the node concept was introduced. Because of this, there are 24 methods that configure the carrier signal directly, but also 24 methods that configure a selectable node. In new user programs, only the latter should be used.
The nodes of an analog output channel can be configured independently. The contribution of nodes can be individually enabled or disabled, which is most useful for the AM and FM nodes. For nodes that are enabled, a number of standard waveform shape functions are available, such as sine, block, triangle, and ramp. These can be modified by controlling their offset, amplitude, frequency, phase, and symmetry; the latter alters the waveform from its regular, symmetrical shape.
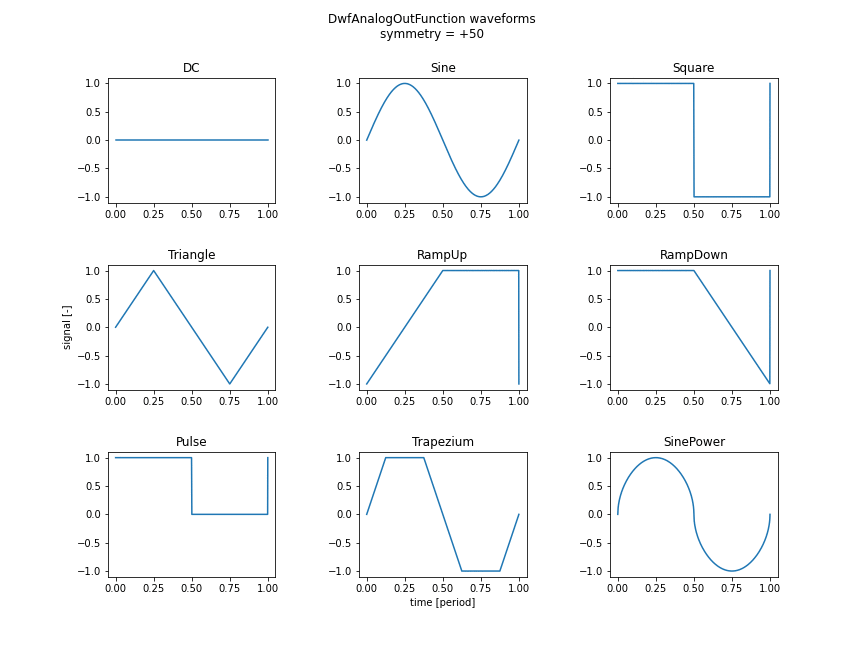
It is also possible to upload an arbitrary wave-shape to the instrument as a sequence of samples to be played. This can be used for short wave-shapes, but it is also possible to perform continuous playback by uploading blocks of samples in a loop.
AnalogOut instrument API overview
With 83 methods, the AnalogOut instrument is the second most complicated instrument supported by the Digilent Waveforms API, after the AnalogIn instrument. Below, we categorize all its methods and shortly introduce them. Detailed information on all methods can be found in the AnalogOut
class reference that follows.
Instrument control
Like all instruments supported by the Digilent Waveforms library, the AnalogOut instrument provides reset()
, configure()
, and status()
methods.
The AnalogOut instrument is unusual in that these methods operate on individual AnalogOut channels, meaning that each AnalogOut channel acts as as a separate, independent instrument.
The reset()
method resets a specific analog output channel (or all channels).
The configure()
method is used to explicitly transfer settings to the instrument, and/or to start a configured operation.
The status()
method retrieves status information from the instrument. It returns the current DwfState
of the AnalogOut instrument.
control operation |
type/unit |
methods |
reset instrument |
n/a |
|
configure instrument |
n/a |
|
request instrument status |
Channel count
This method returns the number of analog output channels.
property |
type/unit |
method |
channel count |
int |
Per-channel state machine settings
These settings determine the duration of the Wait
and Running
states, how many times the Wait/Running cycle should be repeated, and whether a trigger must precede each Wait/Running cycle.
The channel master setting allows an analog output channel to be controlled by another channel, synchronizing their behavior.
setting |
type/unit |
methods |
wait duration |
float [s] |
|
run duration |
float [s] |
|
repeat count |
int [-] |
|
repeat trigger |
bool |
|
channel master |
int |
Per-channel trigger configuration
These settings configure the channel trigger.
setting |
type/unit |
methods |
trigger source |
||
trigger slope |
Note
The triggerSourceInfo()
method is obsolete. Use the generic DwfDevice.triggerInfo()
method instead.
Per-channel output settings
These settings determine the channel output behavior.
setting |
type/unit |
methods |
channel mode |
||
channel idle |
|
|
channel limitation |
float [V] or [A] |
Per-channel miscellaneous settings
The function of the custom AM/FM enable setting is currently not understood. It is only applicable to Electronics Explorer devices, as stated in a message on the Digilent forum.
Todo
Figure out what the custom AM/FM enable setting does.
setting |
type/unit |
methods |
custom AM/FM enable |
bool |
Node enumeration
This method enumerates all nodes of an AnalogOut channel.
property |
type/unit |
methods |
node enumeration |
|
Node configuration
These methods configure the output signal of an AnalogOut channel node.
setting |
type/unit |
methods |
|
node enable |
bool |
||
node function |
|||
node frequency |
float [Hz] |
||
node amplitude |
float [V] |
||
node offset |
float [V] | |
||
node symmetry |
float [%] | |
||
node phase |
float [deg] |
Node data management
These methods transfer arbitrary waveform data to an AnalogOut channel node.
operation |
type/unit |
methods |
node data upload |
n/a |
|
node play status |
n/a |
|
node play data upload |
n/a |
Carrier configuration (obsolete)
Note
These methods have been replaced by equivalent node methods.
setting |
type/unit |
methods |
carrier enable |
bool |
|
carrier function |
||
carrier frequency |
float [Hz] |
|
carrier amplitude |
float [V] |
|
carrier offset |
float [V] |
|
carrier symmetry |
float [%] |
|
carrier phase |
float [deg] |
|
Carrier node data management (obsolete)
Note
These methods have been replaced by equivalent node methods.
operation |
type/unit |
methods |
carrier data upload |
n/a |
|
carrier play status |
n/a |
|
carrier play data upload |
n/a |
AnalogOut reference
- class AnalogOut
The AnalogOut class provides access to the analog output (signal generator) instrument of a
DwfDevice
.Attention
Users of pydwf should not create instances of this class directly.
It is instantiated during initialization of a DwfDevice and subsequently assigned to its public
analogOut
attribute for access by the user.- reset(channel_index: int) None
Reset the AnalogOut instrument.
- Raises:
DwfLibraryError – An error occurred while executing the reset operation.
- configure(channel_index: int, start: int) None
Configure the AnalogOut instrument.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the configure operation.
- status(channel_index: int) DwfState
Get the AnalogOut instrument channel state.
This method performs a status request to the AnalogOut instrument and receives its response.
- Parameters:
channel_index (int) – The output channel for which to get the status.
- Returns:
The status of the AnalogOut instrument channel.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the status operation.
- count() int
Count the number of analog output channels.
- Returns:
The number of analog output channels.
- Return type:
- Raises:
DwfLibraryError – An error occurred while retrieving the number of analog output channels.
- waitInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel valid
Wait
state duration range, in seconds.- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The range of configurable
Wait
state durations, in seconds.- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- waitSet(channel_index: int, wait_duration: float) None
Set the AnalogOut channel
Wait
state duration, in seconds.- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- waitGet(channel_index: int) float
Get the AnalogOut channel
Wait
state duration, in seconds.- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured
Wait
state duration.- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- runInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel valid
Running
state duration range, in seconds.- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The range of allowed
Running
state durations, in seconds.- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- runSet(channel_index: int, run_duration: float) None
Set the AnalogOut channel
Running
state duration, in seconds.- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- runGet(channel_index: int) float
Get the AnalogOut channel
Running
state duration, in seconds.- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured
Running
state duration, in seconds.- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- runStatus(channel_index: int) float
Get
Running
state duration time left.- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The current time remaining in the
Running
state, in seconds.- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- repeatTriggerSet(channel_index: int, repeat_trigger_flag: bool) None
Set the AnalogOut channel repeat trigger setting.
This setting determines if a new trigger must precede all Wait/Running sequences, or only the first one.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- repeatTriggerGet(channel_index: int) bool
Get the AnalogOut channel repeat trigger setting.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured repeat trigger setting.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- repeatInfo(channel_index: int) Tuple[int, int]
Get AnalogOut repeat count range.
The repeat count is the number of times the AnalogOut channel will go through the Wait/Running or Armed/Wait/Running state cycles during the output sequence.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The range of configurable repeat values.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- repeatSet(channel_index: int, repeat: int) None
Set the AnalogOut repeat count.
The repeat count is the number of times the AnalogOut channel will go through the Wait/Running or Armed/Wait/Running state cycles during the output sequence.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- repeatGet(channel_index: int) int
Get the AnalogOut repeat count.
The repeat count is the number of times the AnalogOut channel will go through the Wait/Running or Armed/Wait/Running state cycles during the output sequence.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured repeat value. 0 means: repeat indefinitely.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- repeatStatus(channel_index: int) int
Get the AnalogOut current repeat count, which decreases to 0 while going through Running/Wait state cycles.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The current repeat count value.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- masterSet(channel_index: int, master_channel_index: int) None
Set the AnalogOut channel master.
Sets the state machine master channel of the analog output channel.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while setting the value.
- masterGet(channel_index: int) int
Get the AnalogOut channel master.
- Parameters:
channel_index (int) – The analog output channel for which to get the master channel.
- Returns:
The index of the master channel which the channel is configured to follow.
- Return type:
- Raises:
DwfLibraryError – An error occurred while getting the value.
- triggerSourceInfo() List[DwfTriggerSource]
Get a list of valid AnalogOut instrument trigger sources.
- Returns:
The list of DwfTriggerSource values that can be configured.
- Return type:
List[DwfTriggerSource]
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- triggerSourceSet(channel_index: int, trigger_source: DwfTriggerSource) None
Set the AnalogOut channel trigger source.
- Parameters:
channel_index (int) – The AnalogOut channel.
trigger_source (DwfTriggerSource) – The trigger source to be selected.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- triggerSourceGet(channel_index: int) DwfTriggerSource
Get the currently selected channel trigger source.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently selected channel trigger source.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- triggerSlopeSet(channel_index: int, trigger_slope: DwfTriggerSlope) None
Select the AnalogOut channel trigger slope.
- Parameters:
channel_index (int) – The AnalogOut channel.
trigger_slope (DwfTriggerSlope) – The trigger slope to be selected.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- triggerSlopeGet(channel_index: int) DwfTriggerSlope
Get the currently selected AnalogOut channel trigger slope.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently selected AnalogOut channel trigger slope.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- modeSet(channel_index: int, mode: DwfAnalogOutMode) None
Set the AnalogOut channel mode (voltage or current).
- Parameters:
channel_index (int) – The AnalogOut channel.
mode (DwfAnalogOutMode) – The analog output mode to configure.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- modeGet(channel_index: int) DwfAnalogOutMode
Get the AnalogOut channel mode (voltage or current).
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured analog output mode.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- idleInfo(channel_index: int) List[DwfAnalogOutIdle]
Get the valid AnalogOut channel idle settings.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
A list of options for the channel behavior when idle.
- Return type:
List[DwfAnalogOutIdle]
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- idleSet(channel_index: int, idle: DwfAnalogOutIdle) None
Set the AnalogOut channel idle behavior.
- Parameters:
channel_index (int) – The AnalogOut channel.
idle (DwfAnalogOutIdle) – The idle behavior setting to be configured.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- idleGet(channel_index: int) DwfAnalogOutIdle
Get the AnalogOut channel idle behavior.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The AnalogOut channel idle behavior setting.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- limitationInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel limitation range, in Volts or Amps.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The range of limitation values that can be configured.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- limitationSet(channel_index: int, limitation: float) None
Set the AnalogOut channel limitation value, in Volts or Amps.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- limitationGet(channel_index: int) float
Get the AnalogOut channel limitation value, in Volts or Amps.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured limitation value, in Volts or Amps.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- customAMFMEnableSet(channel_index: int, enable: bool) None
Set the AnalogOut channel custom AM/FM enable status.
Todo
Understand and document what this setting does.
Note
This setting is only applicable to Electronics Explorer devices, as stated in a message on the Digilent forum.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- customAMFMEnableGet(channel_index: int) bool
Get the AnalogOut channel custom AM/FM enable status.
Todo
Understand and document what this setting does.
Note
This setting is only applicable to Electronics Explorer devices, as stated in a message on the Digilent forum.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The custom AM/FM enable state.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeInfo(channel_index: int) List[DwfAnalogOutNode]
Get a list of valid AnalogOut channel nodes.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The valid nodes for this channel.
- Return type:
List[DwfAnalogOutNode]
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeEnableSet(channel_index: int, node: DwfAnalogOutNode, mode: int) None
Enabled or disable an AnalogOut channel node.
The carrier node enables or disables the channel or selects the modulation. With channel_index -1, each analog-out channel enable mode will be configured to the same, new option.
- Parameters:
channel_index (int) – The AnalogOut channel. Specify -1 to configure all AnalogOut channels.
node (DwfAnalogOutNode) – The channel node.
mode (int) – The enable mode.
Note
The precise meaning of the mode parameter is not clear from the documentation.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeEnableGet(channel_index: int, node: DwfAnalogOutNode) int
Get the enabled state of an AnalogOut channel node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The currently configured enable mode setting.
- Return type:
Note
The precise meaning of the mode parameter is not clear from the documentation.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeFunctionInfo(channel_index: int, node: DwfAnalogOutNode) List[DwfAnalogOutFunction]
Get the valid waveform shape function options of an AnalogOut channel node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The available node waveform shape functions.
- Return type:
List[DwfAnalogOutFunction]
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeFunctionSet(channel_index: int, node: DwfAnalogOutNode, func: DwfAnalogOutFunction) None
Set the waveform shape function for an AnalogOut channel node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
func (DwfAnalogOutFunction) – The waveform shape function to be configured.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeFunctionGet(channel_index: int, node: DwfAnalogOutNode) DwfAnalogOutFunction
Get the waveform shape function for an AnalogOut channel node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The currently configured waveform shape function.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeFrequencyInfo(channel_index: int, node: DwfAnalogOutNode) Tuple[float, float]
Get the channel node valid frequency range for an AnalogOut channel node, in Hz.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The range of valid frequencies, in Hz.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeFrequencySet(channel_index: int, node: DwfAnalogOutNode, frequency: float) None
Set the channel node frequency for an AnalogOut channel node, in Hz.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
frequency (float) – The frequency, in Hz.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeFrequencyGet(channel_index: int, node: DwfAnalogOutNode) float
Get the frequency for an AnalogOut channel node, in Hz.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The currently configured frequency, in Hz.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeAmplitudeInfo(channel_index: int, node: DwfAnalogOutNode) Tuple[float, float]
Get the amplitude range for an AnalogOut channel node, in Volts.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The range of allowed amplitude values, in Volts.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeAmplitudeSet(channel_index: int, node: DwfAnalogOutNode, amplitude: float) None
Set the amplitude for an AnalogOut channel node, in Volts.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
amplitude (float) – The amplitude to be configured, in Volts.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeAmplitudeGet(channel_index: int, node: DwfAnalogOutNode) float
Get the amplitude for an AnalogOut channel node, in Volts.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The currently configured amplitude, in Volts.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeOffsetInfo(channel_index: int, node: DwfAnalogOutNode) Tuple[float, float]
Get the valid offset range for an AnalogOut channel node, in Volts.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The range of valid node offsets, in Volts.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeOffsetSet(channel_index: int, node: DwfAnalogOutNode, offset: float) None
Set the offset for an AnalogOut channel node, in Volts.
Note
Configuring the offset of the Carrier node takes a noticeable amount of time (100s of milliseconds).
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
offset (float) – The channel offset to be configured, in Volts.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeOffsetGet(channel_index: int, node: DwfAnalogOutNode) float
Get the offset for an AnalogOut channel node, in Volts.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The currently configured node offset.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeSymmetryInfo(channel_index: int, node: DwfAnalogOutNode) Tuple[float, float]
Get the symmetry range for an AnalogOut channel node.
The symmetry value alters the waveform shape function of the node.
The symmetry value ranges from 0 to 100 for most waveform shape functions, except for the
SinePower
waveform shape function, where it ranges from -100 to +100.- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The range of valid symmetry settings.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeSymmetrySet(channel_index: int, node: DwfAnalogOutNode, symmetry: float) None
Set the symmetry value for an AnalogOut channel node.
The symmetry value alters the waveform shape function of the node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
symmetry (float) – The symmetry setting.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeSymmetryGet(channel_index: int, node: DwfAnalogOutNode) float
Get the symmetry value for an AnalogOut channel node.
The symmetry value alters the waveform shape function of the node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The currently configured channel node symmetry value.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodePhaseInfo(channel_index: int, node: DwfAnalogOutNode) Tuple[float, float]
Get the valid phase range for an AnalogOut channel node, in degrees.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The range of valid channel node phase values, in degrees.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodePhaseSet(channel_index: int, node: DwfAnalogOutNode, phase: float) None
Set the phase for an AnalogOut channel node, in degrees.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
phase (float) – The phase setting, in degrees.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodePhaseGet(channel_index: int, node: DwfAnalogOutNode) float
Get the phase for an AnalogOut channel node, in degrees.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The currently configured node phase value, in degrees.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeDataInfo(channel_index: int, node: DwfAnalogOutNode) Tuple[float, float]
Get data range for an AnalogOut channel node, in samples.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The range of valid values.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodeDataSet(channel_index: int, node: DwfAnalogOutNode, data: ndarray) None
Set the data for an AnalogOut channel node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
data (np.ndarray) – The data.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodePlayStatus(channel_index: int, node: DwfAnalogOutNode) Tuple[int, int, int]
Get the play status for an AnalogOut channel node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
- Returns:
The free, lost, and corrupted status counts, in samples.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- nodePlayData(channel_index: int, node: DwfAnalogOutNode, data: ndarray) None
Provide the playback data for an AnalogOut channel node.
- Parameters:
channel_index (int) – The AnalogOut channel.
node (DwfAnalogOutNode) – The channel node.
data (np.ndarray) – The playback data.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- enableSet(channel_index: int, enable: bool) None
Enable or disable the specified AnalogOut channel.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- enableGet(channel_index: int) bool
Get the current enable/disable status of the specified AnalogOut channel.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The ‘enable’ state of the channel.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- functionInfo(channel_index: int) List[DwfAnalogOutFunction]
Get the AnalogOut channel waveform shape function info.
- Returns:
The valid waveform shape functions.
- Return type:
List[DwfAnalogOutFunction]
- Parameters:
channel_index (int) – The AnalogOut channel.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- functionSet(channel_index: int, func: DwfAnalogOutFunction) None
Set the AnalogOut channel waveform shape function.
- Parameters:
channel_index (int) – The AnalogOut channel.
func (DwfAnalogOutFunction) – The waveform shape function to use.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- functionGet(channel_index: int) DwfAnalogOutFunction
Get the AnalogOut channel waveform shape function.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured waveform shape function.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- frequencyInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel valid frequency range, in Hz.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The valid frequency range, in Hz.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- frequencySet(channel_index: int, frequency: float) None
Set the AnalogOut channel frequency, in Hz.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- frequencyGet(channel_index: int) float
Get the AnalogOut channel frequency, in Hz.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured frequency, in Hz.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- amplitudeInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel amplitude range info.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The range of valid amplitudes, in Volts.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- amplitudeSet(channel_index: int, amplitude: float) None
Set the AnalogOut channel amplitude.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- amplitudeGet(channel_index: int) float
Get the AnalogOut channel amplitude.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured amplitude, in Volts.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- offsetInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel offset range info, in Volts.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The valid range of offset values, in Volts.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- offsetSet(channel_index: int, offset: float) None
Set the AnalogOut channel offset, in Volts.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- offsetGet(channel_index: int) float
Get the AnalogOut channel offset, in Volts.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The valid offset value, in Volts.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- symmetryInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel symmetry setting range.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The range of valid symmetry settings.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- symmetrySet(channel_index: int, symmetry: float) None
Set the AnalogOut channel symmetry setting.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- symmetryGet(channel_index: int) float
Get the AnalogOut channel symmetry setting.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured symmetry setting.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- phaseInfo(channel_index: int) Tuple[float, float]
Get the AnalogOut channel phase range, in degrees.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The range of valid phase values, in degrees.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- phaseSet(channel_index: int, phase: float) None
Set the AnalogOut channel phase, in degrees.
- Parameters:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- phaseGet(channel_index: int) float
Get the AnalogOut channel phase, in degrees.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The currently configured phase, in degrees.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- dataInfo(channel_index: int) Tuple[int, int]
Get the AnalogOut channel data buffer range.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The data range.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- dataSet(channel_index: int, data: ndarray) None
Set the AnalogOut channel data.
- Parameters:
channel_index (int) – The AnalogOut channel.
data (np.ndarray) – The data.
- Raises:
DwfLibraryError – An error occurred while executing the operation.
- playStatus(channel_index: int) Tuple[int, int, int]
Get the AnalogOut channel playback status, in samples.
- Parameters:
channel_index (int) – The AnalogOut channel.
- Returns:
The playback status.
- Return type:
- Raises:
DwfLibraryError – An error occurred while executing the operation.